Recipe 1: CSS Animations - shooting stars passing by
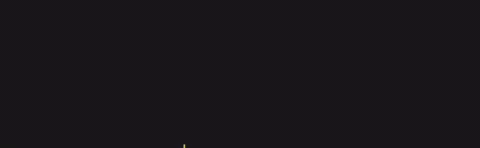
01. Get started
Begin by creating a folder to store the files. Create an additional folder within it called css to store the styling files. The HTML file will be stored in the root folder.
02. Create the page structure
Open your code editor and create an index.html document to contain mark up for the main web page. Begin by creating the basic structure and give a suitable title to the page.
Shooting stars passing by
03. Create the shooting stars
We are going to create two shooting stars so we will need one div for each and a container div. Following code goes inside the body tag.
Now let's style our shooting stars. Following code needs to go into the styles.css file.
.pulses {
position: absolute;
top: 0;
left: 0;
right: 0;
height: 100%;
margin: auto;
width: 45vw;
}
.pulses .up, .pulses .down {
position: absolute;
width: 2px;
height: 100%;
top: 0;
left: 50%;
overflow: hidden;
}
04. Animation definitions
Start with the downward motion.
.pulses .down::before{
content: "";
display: block;
position: absolute;
height: 15vh;
width: 100%;
top: -50%;
left: 0;
background: linear-gradient(to bottom, rgba(255, 255, 255, 0) 0%, #FFD700 75%, #FFD700 100%);
-webkit-animation: down 7s 0s infinite;
animation: down 7s 0s infinite;
-webkit-animation-fill-mode: backwards;
animation-fill-mode: backwards;
-webkit-animation-timing-function: cubic-bezier(0.4, 0.26, 0, 0.97);
animation-timing-function: cubic-bezier(0.4, 0.26, 0, 0.97);
}
@keyframes down {
0% {
top: -50%;
}
100% {
top: 100%;
}
}
05. Et voilà
See the Pen GRRbXrv by sugar2code (@sugar2code) on CodePen.